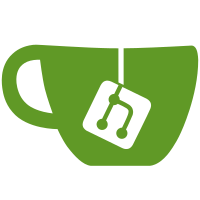
Also added some 5-second timeouts in various places to build.xml because (expected) exceptions were causing ant to hang for a long time, then inexplicably continue.
783 lines
20 KiB
XML
783 lines
20 KiB
XML
<?xml version="1.0" ?>
|
|
|
|
<project
|
|
basedir="."
|
|
default="run"
|
|
name="Thinking in Java, 4th Edition (Refreshed) by Bruce Eckel (chapter: concurrency)">
|
|
|
|
<description>
|
|
build.xml for the source code for the concurrency chapter of
|
|
Thinking in Java, 4th Edition (Refreshed) by Bruce Eckel
|
|
Source code available at http://www.MindView.net
|
|
See copyright notice in CopyRight.txt
|
|
|
|
Ant available from: http://ant.apache.org/
|
|
|
|
To see options, type: ant -p
|
|
</description>
|
|
|
|
<condition property="version1.8">
|
|
<equals arg1="1.8" arg2="${ant.java.version}"/>
|
|
</condition>
|
|
|
|
<target name="net_mindview_util">
|
|
<javac includeantruntime="false"
|
|
classpath="${basedir}/.."
|
|
srcdir="${basedir}/../net/mindview/util/">
|
|
<compilerarg value="-Xmaxerrs"/>
|
|
<compilerarg value="10"/>
|
|
</javac>
|
|
</target>
|
|
|
|
<target name="enumerated_menu">
|
|
<javac includeantruntime="false"
|
|
classpath="${basedir}/.."
|
|
srcdir="${basedir}/../enumerated/menu/">
|
|
<compilerarg value="-Xmaxerrs"/>
|
|
<compilerarg value="10"/>
|
|
</javac>
|
|
</target>
|
|
|
|
<target
|
|
depends="net_mindview_util,enumerated_menu"
|
|
description="Build all classes in this directory"
|
|
name="build">
|
|
<fail message="J2SE8 required" unless="version1.8"/>
|
|
<echo message="Building 'concurrency'"/>
|
|
<javac includeantruntime="false"
|
|
classpath="${basedir}/.."
|
|
debug="true"
|
|
srcdir="${basedir}">
|
|
<compilerarg value="-Xmaxerrs"/>
|
|
<compilerarg value="10"/>
|
|
</javac>
|
|
<echo message="Build 'concurrency' succeeded"/>
|
|
</target>
|
|
|
|
<target name="ActiveObjectDemo">
|
|
<java
|
|
classname="ActiveObjectDemo"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="AtomicEvenGenerator">
|
|
<java
|
|
classname="AtomicEvenGenerator"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="AtomicIntegerTest">
|
|
<java
|
|
classname="AtomicIntegerTest"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="AtomicityTest">
|
|
<java
|
|
classname="AtomicityTest"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="AttemptLocking">
|
|
<java
|
|
classname="AttemptLocking"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="BankTellerSimulation">
|
|
<java
|
|
classname="BankTellerSimulation"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true">
|
|
<arg line="5"/>
|
|
</java>
|
|
</target>
|
|
|
|
<target name="BasicThreads">
|
|
<java
|
|
classname="BasicThreads"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="CachedThreadPool">
|
|
<java
|
|
classname="CachedThreadPool"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="CallableDemo">
|
|
<java
|
|
classname="CallableDemo"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="CaptureUncaughtException">
|
|
<java
|
|
classname="CaptureUncaughtException"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="false"
|
|
fork="true"
|
|
timeout="5000"
|
|
/>
|
|
</target>
|
|
|
|
<target name="CarBuilder">
|
|
<java
|
|
classname="CarBuilder"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="CloseResource">
|
|
<java
|
|
classname="CloseResource"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="CountDownLatchDemo">
|
|
<java
|
|
classname="CountDownLatchDemo"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="CriticalSection">
|
|
<java
|
|
classname="concurrency.CriticalSection"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="DaemonFromFactory">
|
|
<java
|
|
classname="DaemonFromFactory"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="Daemons">
|
|
<java
|
|
classname="Daemons"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="DaemonsDontRunFinally">
|
|
<java
|
|
classname="DaemonsDontRunFinally"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="DeadlockingDiningPhilosophers">
|
|
<java
|
|
classname="DeadlockingDiningPhilosophers"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true">
|
|
<arg line="0 5 timeout"/>
|
|
</java>
|
|
</target>
|
|
|
|
<target name="DelayQueueDemo">
|
|
<java
|
|
classname="DelayQueueDemo"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="EvenGenerator">
|
|
<java
|
|
classname="EvenGenerator"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="ExceptionThread">
|
|
<java
|
|
classname="ExceptionThread"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="false"
|
|
fork="true"
|
|
timeout="5000" />
|
|
<echo message="* Exception was expected *"/>
|
|
</target>
|
|
|
|
<target name="ExchangerDemo">
|
|
<java
|
|
classname="ExchangerDemo"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="ExplicitCriticalSection">
|
|
<java
|
|
classname="concurrency.ExplicitCriticalSection"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="FastSimulation">
|
|
<java
|
|
classname="FastSimulation"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="FixedDiningPhilosophers">
|
|
<java
|
|
classname="FixedDiningPhilosophers"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true">
|
|
<arg line="5 5 timeout"/>
|
|
</java>
|
|
</target>
|
|
|
|
<target name="FixedThreadPool">
|
|
<java
|
|
classname="FixedThreadPool"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="GreenhouseScheduler">
|
|
<java
|
|
classname="GreenhouseScheduler"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true">
|
|
<arg line="5000"/>
|
|
</java>
|
|
</target>
|
|
|
|
<target name="HorseRace">
|
|
<java
|
|
classname="HorseRace"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="Interrupting">
|
|
<java
|
|
classname="Interrupting"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="Interrupting2">
|
|
<java
|
|
classname="Interrupting2"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="InterruptingIdiom">
|
|
<java
|
|
classname="InterruptingIdiom"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true">
|
|
<arg line="1100"/>
|
|
</java>
|
|
</target>
|
|
|
|
<target name="Joining">
|
|
<java
|
|
classname="Joining"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="ListComparisons">
|
|
<java
|
|
classname="ListComparisons"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true">
|
|
<arg line="1 10 10"/>
|
|
</java>
|
|
</target>
|
|
|
|
<target name="MainThread">
|
|
<java
|
|
classname="MainThread"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="MapComparisons">
|
|
<java
|
|
classname="MapComparisons"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true">
|
|
<arg line="1 10 10"/>
|
|
</java>
|
|
</target>
|
|
|
|
<target name="MoreBasicThreads">
|
|
<java
|
|
classname="MoreBasicThreads"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="MultiLock">
|
|
<java
|
|
classname="MultiLock"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="MutexEvenGenerator">
|
|
<java
|
|
classname="MutexEvenGenerator"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="NaiveExceptionHandling">
|
|
<java
|
|
classname="NaiveExceptionHandling"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="false"
|
|
fork="true"
|
|
timeout="5000"/>
|
|
<echo message="* Exception was expected *"/>
|
|
</target>
|
|
|
|
<target name="NIOInterruption">
|
|
<java
|
|
classname="NIOInterruption"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="NotifyVsNotifyAll">
|
|
<java
|
|
classname="NotifyVsNotifyAll"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="OrnamentalGarden">
|
|
<java
|
|
classname="OrnamentalGarden"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="PipedIO">
|
|
<java
|
|
classname="PipedIO"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="PriorityBlockingQueueDemo">
|
|
<java
|
|
classname="PriorityBlockingQueueDemo"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="ReaderWriterList">
|
|
<java
|
|
classname="ReaderWriterList"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="ResponsiveUI">
|
|
<java
|
|
classname="ResponsiveUI"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="Restaurant">
|
|
<java
|
|
classname="Restaurant"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="SelfManaged">
|
|
<java
|
|
classname="SelfManaged"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="SemaphoreDemo">
|
|
<java
|
|
classname="SemaphoreDemo"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="SerialNumberChecker">
|
|
<java
|
|
classname="SerialNumberChecker"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true">
|
|
<arg line="4"/>
|
|
</java>
|
|
</target>
|
|
|
|
<target name="SettingDefaultHandler">
|
|
<java
|
|
classname="SettingDefaultHandler"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="false"
|
|
fork="true"
|
|
timeout="5000"/>
|
|
</target>
|
|
|
|
<target name="SimpleDaemons">
|
|
<java
|
|
classname="SimpleDaemons"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="SimpleMicroBenchmark">
|
|
<java
|
|
classname="SimpleMicroBenchmark"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="SimplePriorities">
|
|
<java
|
|
classname="SimplePriorities"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="SimpleThread">
|
|
<java
|
|
classname="SimpleThread"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="SingleThreadExecutor">
|
|
<java
|
|
classname="SingleThreadExecutor"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="SleepingTask">
|
|
<java
|
|
classname="SleepingTask"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="SynchronizationComparisons">
|
|
<java
|
|
classname="SynchronizationComparisons"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="SynchronizedEvenGenerator">
|
|
<java
|
|
classname="SynchronizedEvenGenerator"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="SyncObject">
|
|
<java
|
|
classname="SyncObject"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="TestBlockingQueues">
|
|
<java
|
|
classname="TestBlockingQueues"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="ThreadLocalVariableHolder">
|
|
<java
|
|
classname="ThreadLocalVariableHolder"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="ThreadVariations">
|
|
<java
|
|
classname="ThreadVariations"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="ToastOMatic">
|
|
<java
|
|
classname="ToastOMatic"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="RestaurantWithQueues">
|
|
<java
|
|
classname="concurrency.restaurant2.RestaurantWithQueues"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/restaurant2/"
|
|
failonerror="true"
|
|
fork="true">
|
|
<arg line="5"/>
|
|
</java>
|
|
</target>
|
|
|
|
<target name="WaxOMatic">
|
|
<java
|
|
classname="concurrency.waxomatic.WaxOMatic"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/waxomatic/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="WaxOMatic2">
|
|
<java
|
|
classname="concurrency.waxomatic2.WaxOMatic2"
|
|
classpath="${java.class.path};${basedir};${basedir}/.."
|
|
dir="../concurrency/waxomatic2/"
|
|
failonerror="true"
|
|
fork="true"/>
|
|
</target>
|
|
|
|
<target name="Atomicity">
|
|
<exec executable="javap">
|
|
<arg value="-c"/>
|
|
<arg value="Atomicity"/>
|
|
</exec>
|
|
</target>
|
|
|
|
<target
|
|
depends="build"
|
|
description="Compile and run"
|
|
name="run">
|
|
<touch file="failures"/>
|
|
<antcall target="ActiveObjectDemo"/>
|
|
<antcall target="AtomicIntegerTest"/>
|
|
<antcall target="AtomicityTest"/>
|
|
<antcall target="AttemptLocking"/>
|
|
<antcall target="BankTellerSimulation"/>
|
|
<antcall target="BasicThreads"/>
|
|
<antcall target="CachedThreadPool"/>
|
|
<antcall target="CallableDemo"/>
|
|
<antcall target="CaptureUncaughtException"/>
|
|
<antcall target="CarBuilder"/>
|
|
<antcall target="CountDownLatchDemo"/>
|
|
<antcall target="CriticalSection"/>
|
|
<antcall target="DaemonFromFactory"/>
|
|
<antcall target="Daemons"/>
|
|
<antcall target="DaemonsDontRunFinally"/>
|
|
<antcall target="DeadlockingDiningPhilosophers"/>
|
|
<antcall target="DelayQueueDemo"/>
|
|
<antcall target="EvenGenerator"/>
|
|
<antcall target="ExceptionThread"/>
|
|
<antcall target="ExchangerDemo"/>
|
|
<antcall target="ExplicitCriticalSection"/>
|
|
<antcall target="FastSimulation"/>
|
|
<antcall target="FixedDiningPhilosophers"/>
|
|
<antcall target="FixedThreadPool"/>
|
|
<antcall target="GreenhouseScheduler"/>
|
|
<antcall target="HorseRace"/>
|
|
<antcall target="Interrupting"/>
|
|
<antcall target="Interrupting2"/>
|
|
<antcall target="InterruptingIdiom"/>
|
|
<antcall target="Joining"/>
|
|
<antcall target="ListComparisons"/>
|
|
<antcall target="MainThread"/>
|
|
<antcall target="MapComparisons"/>
|
|
<antcall target="MoreBasicThreads"/>
|
|
<antcall target="MultiLock"/>
|
|
<antcall target="NaiveExceptionHandling"/>
|
|
<antcall target="NIOInterruption"/>
|
|
<antcall target="NotifyVsNotifyAll"/>
|
|
<antcall target="OrnamentalGarden"/>
|
|
<antcall target="PipedIO"/>
|
|
<antcall target="PriorityBlockingQueueDemo"/>
|
|
<antcall target="ReaderWriterList"/>
|
|
<antcall target="Restaurant"/>
|
|
<antcall target="SelfManaged"/>
|
|
<antcall target="SemaphoreDemo"/>
|
|
<antcall target="SerialNumberChecker"/>
|
|
<antcall target="SettingDefaultHandler"/>
|
|
<antcall target="SimpleDaemons"/>
|
|
<antcall target="SimpleMicroBenchmark"/>
|
|
<antcall target="SimplePriorities"/>
|
|
<antcall target="SimpleThread"/>
|
|
<antcall target="SingleThreadExecutor"/>
|
|
<antcall target="SleepingTask"/>
|
|
<antcall target="SynchronizationComparisons"/>
|
|
<antcall target="SyncObject"/>
|
|
<antcall target="ThreadLocalVariableHolder"/>
|
|
<antcall target="ThreadVariations"/>
|
|
<antcall target="ToastOMatic"/>
|
|
<antcall target="RestaurantWithQueues"/>
|
|
<antcall target="WaxOMatic"/>
|
|
<antcall target="WaxOMatic2"/>
|
|
<antcall target="Atomicity"/>
|
|
<echo
|
|
message="* AtomicEvenGenerator must be run by hand *"/>
|
|
<echo message="* CloseResource must be run by hand *"/>
|
|
<echo message="* MutexEvenGenerator must be run by hand *"/>
|
|
<echo message="* ResponsiveUI must be run by hand *"/>
|
|
<echo
|
|
message="* SynchronizedEvenGenerator must be run by hand *"/>
|
|
<echo message="* TestBlockingQueues must be run by hand *"/>
|
|
<delete file="failures"/>
|
|
</target>
|
|
|
|
<target description="delete all byproducts" name="clean">
|
|
<delete>
|
|
<fileset dir="${basedir}" includes="**/*.class"/>
|
|
<fileset dir="${basedir}" includes="**/*Output.txt"/>
|
|
<fileset dir="${basedir}" includes="**/log.txt"/>
|
|
<fileset dir="${basedir}" includes="failures"/>
|
|
</delete>
|
|
<echo message="clean successful"/>
|
|
</target>
|
|
|
|
</project>
|
|
|
|
|